이 글을 이해한다면 스프링 시큐리시의 간단한 개념과 아래와 같은 형태의 세팅 방법에 대해 알 수 있다!
- URL 별로 접근 권한 처리를 하고 싶은 경우
- 로그인한 사용자와 관리자 권한을 가진 사용자가 지정한 리소스만 접근 가능하도록 세팅하고 싶은 경우
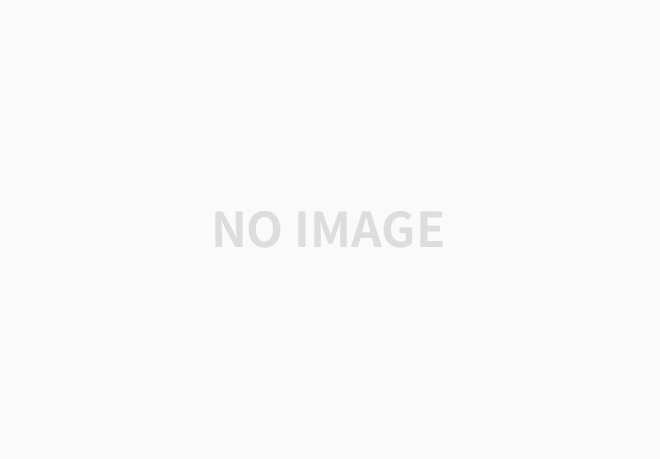
Spring Security란?
Spring Security is a powerful and highly customizable authentication and access-control framework. It is the de-facto standard for securing Spring-based applications.
Spring Security is a framework that focuses on providing both authentication and authorization to Java applications. Like all Spring projects, the real power of Spring Security is found in how easily it can be extended to meet custom requirements
- 출처 : spring 공식 사이트 https://spring.io/projects/spring-security
- 공식 사이트의 기준 정의
- Spring Security는 사용자 정의가 가능한 인증 및 액세스 제어 프레임워크
- 스프링 기반 애플리케이션 보안을 위한 표준
- 자바 애플리케이션에 인증과 인가(권한 부여)를 모두 제공하는데 중점을 둔 프레임 워크
- 특징
- 인증 및 인가에 대한 포괄적이고 확장 가능한 지원
- 세션 고정, 크로스 사이트 요청 위조 등과 같은 공격으로 부터 보호 등
쉽게 말해서 스프링으로 개발된 애플리케이션을 모두가 접근하고 사용하는 것이 아니라 필요에 따라 본인을 확인하거나, 접근 가능한 정보 권한을 부여하는 것을 편하게 할 수 있도록하는 프레임워크라고 보면 된다.
- 인증 Authentication : 증명, 자격 증명 검증으로 본인 확인을 하는 것으로 보통 ID, Password로 인증함
- 인가 Authorization : 인증 이후 접근 권한을 결정하는 절차로, 부여된 접근 권한에만 접근이 가능함
- 예) 관리자페이지는 ADMIN 권한을 가진 사용자만 접근이 가능함
Spring Security 용어
- Authentication : 인증, 사용자 본인 확인 절차
- Authorization : 인가, 인증된 사용자가 요청한 리소스에 접근 가능한지 확인
- Principal : 접근 주체, 보호된 리소스에 접근하는 사용자
- Role : 권한, 인증된 사용자가 애플리케이션의 동작을 수행할 수 있도록 허락되어 있는지 결정
- 웹 요청 권한 or 메서드 호출 및 도메인 인스턴스 접근 권한 부여가 있음
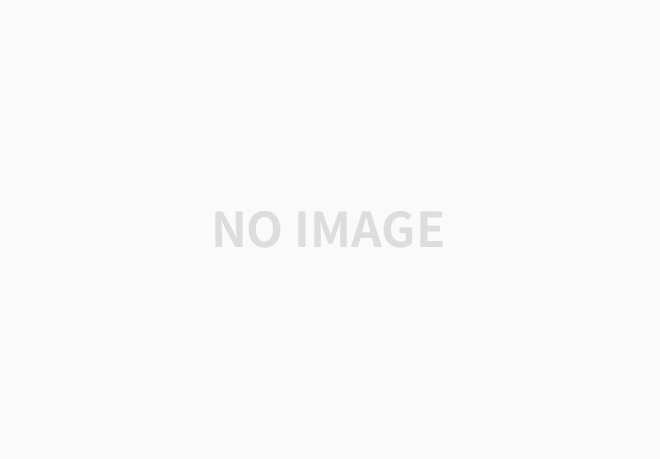
Spring Security 세팅
build.gradle 설정
스프링 시큐리티 관련 dependencies 추가
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-security'
testImplementation 'org.springframework.security:spring-security-test'
}
스프링 시큐리티 라이브러리를 추가하고 서버를 띄우면 아래와 같은 화면이 나옴!
스프링 시큐리티는 기본적으로 인증되지 않은 사용자는 서비스를 사용할 수 없게 되어 있다.
시큐리티 관련 설정을 하면 아래 로그인 화면은 보이지 않게 된다.
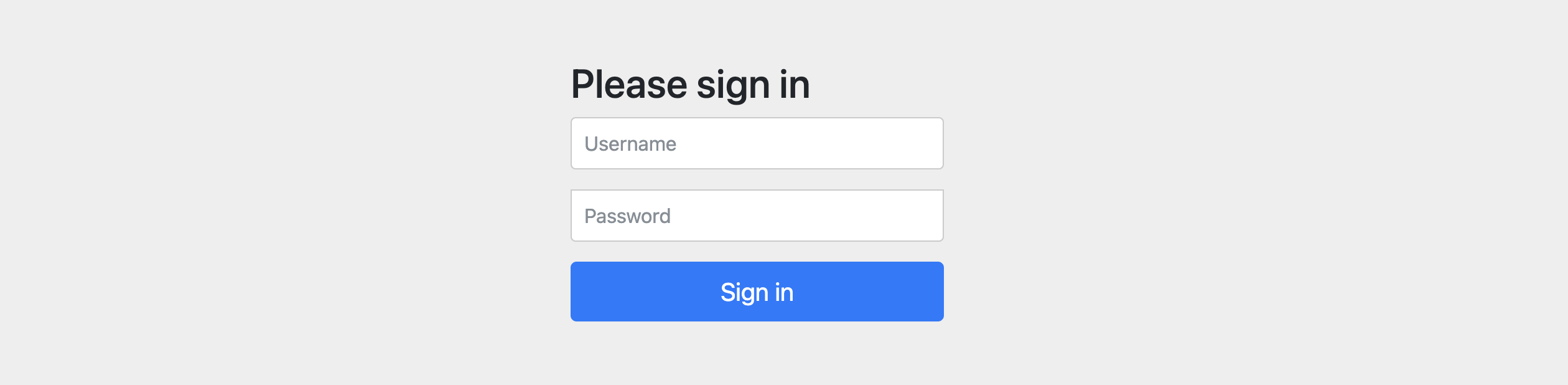
위 화면에서 로그인 하기 위해서는 서버 띄운 콘솔에서 아래 password를 입력하면 된다.
(아래 방식으로 로그인 하는건 개발용으로만 사용하고, config 세팅을 하면 해당 화면이 사라짐)

Using generated security password: f85c2388-b519-482b-9218-10399417733c
This generated password is for development use only. Your security configuration must be updated before running your application in production.
Username에는 user 입력, 콘솔에서 확인한 비번을 입력하면 로그인 가능
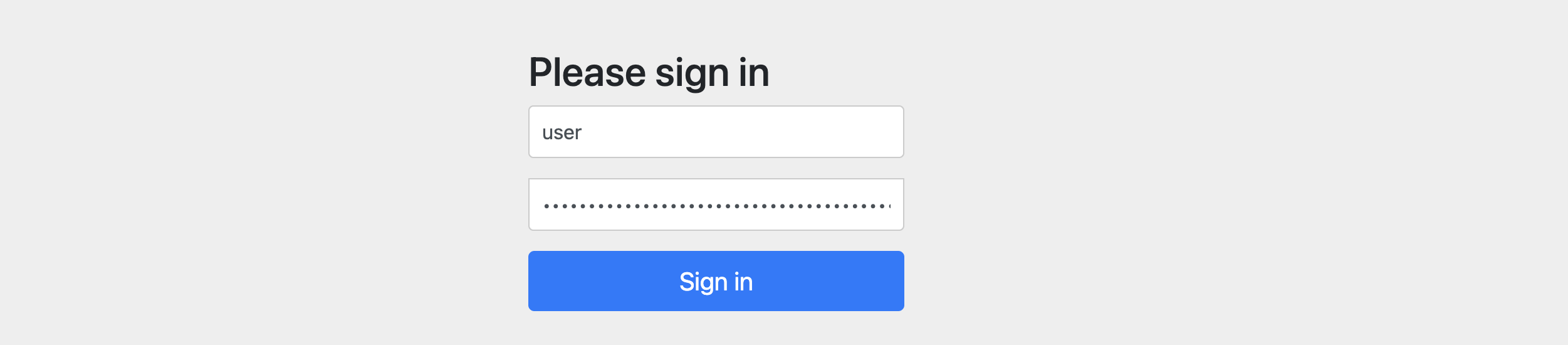
WebSecurityConfing 세팅
스프링 시큐리티 라이브러리를 추가 한 후 아래 설정을 추가 하면 된다.
아래와 같이 사용자가 원하는 대로 설정을 해서 인증 사용자, 권한 부여 사용자 관련 설정을 할 수 있다.
@EnableWebSecurity : 모든 요청 URL이 스프링 시큐리티의 제어를 받게함, 시큐리티 필터 등록 됨
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.method.configuration.EnableGlobalMethodSecurity;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.web.SecurityFilterChain;
@Configuration
@EnableWebSecurity // 스프링 시큐리티 필터가 스프링 필터체인에 등록이 됨
@EnableGlobalMethodSecurity(securedEnabled = true, prePostEnabled = true) // @secured 활성화
public class WebSecurityConfig {
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/user/**").authenticated() // /user path는 인증된 사용자만 접근 가능
.antMatchers("/manager/**").hasAnyRole("ADMIN", "MANAGER") // /manager path는 ADMIN과 MANAGER 권한 가진 사용자만 접근 가능
.antMatchers("/admin/**").hasRole("ADMIN") // /admin path는 ADMIN 권한 가진 사용자만 접근 가능
.anyRequest().permitAll(); // 다른 모든 요청들은 인증이나 권한 없이 허용
return http.build();
}
}
스프링 시큐리티를 설정하기 전에 위와 같이 path 설계, 권한 설계가 먼저 진행 되어야 한다.
위와 같이 설계 하는 경우 아래와 같은 형태가 된다.
- /user 시작되는 path 접근 시
- 인증 된 사용자만 접근 가능(로그인 된 사용자만!), 로그인 하지 않은 경우 403 내려감
- /manager 시작되는 path 접근 시
- 로그인 후 사용자의 권한이 ADMIN 또는 MANAGER 인 사용자만 접근 가능
- 일반 사용자는 로그인 하더라도 접근 불가
- /admin 시작되는 path 접근 시
- 로그인 후 사용자의 권한이 ADMIN인 사용자만 접근 가능
- MANAGER or 일반 사용자 모두 접근 불가
'Java | spring > Spring Security' 카테고리의 다른 글
필터 기반으로 동작하는 Spring Security & 필터 종류 (0) | 2023.10.02 |
---|
댓글