스프링 시큐리티는 필터 기반으로 동작한다.
하여 스프링 시큐리티를 이해하기 위해서는 필터를 제대로 이해하는 것이 좋다!
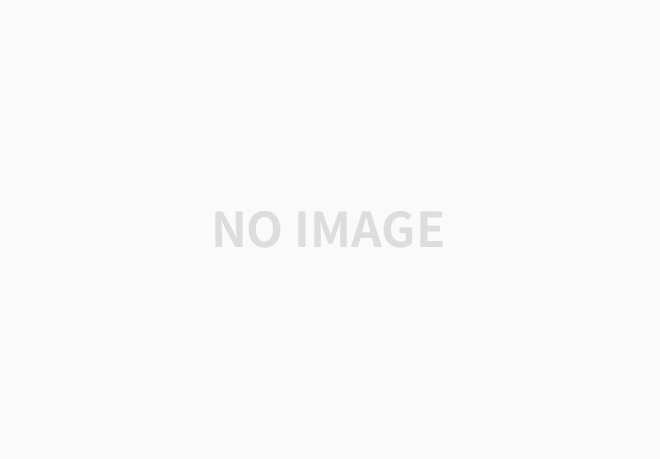
필터(Filter) 란 무엇인가?
- 필터는 요청과 응답을 걸려서 정제하는 역할
- 클라이언트로부터 오는 request/response에 대해 최초,최종 단계에 존재
- 사용되는 용도 : 로깅 용도, 인증, 권한 체크 등
- ex) 들어온 요청이 DispatcherServlet에 전달되기 전에 헤더를 검사해 인증 토큰 여부, 변조 검사 등을 함
- 여러개의 필터가 모여서 하나의 체인(chain)을 형성 할 수 있음
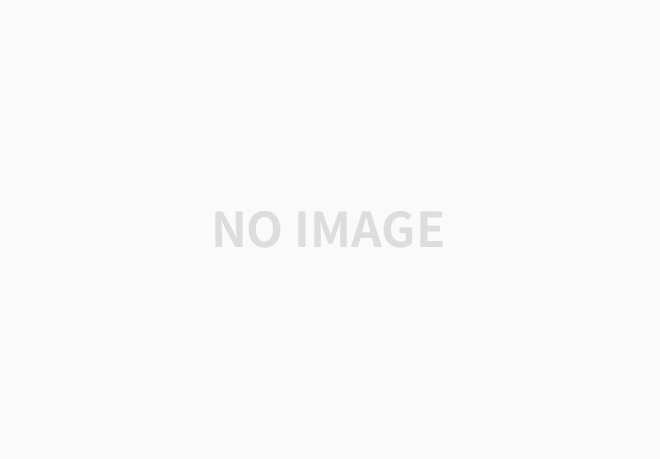
- filterchain 사용 코드
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) {
// do something before the rest of the application
chain.doFilter(request, response); // invoke the rest of the application
// do something after the rest of the application
}
Spring Security Filter란?
- 스프링 시큐리티 필터는 다양한 필터들로 나누어져 있으며, 각각 인증, 인가 관련 작업 처리
- 기본적으로 세팅된 필터 실행 순서가 있으나 필요시에는 사용자 커스텀 필터를 추가하거나, 특정 필터 삭제 가능
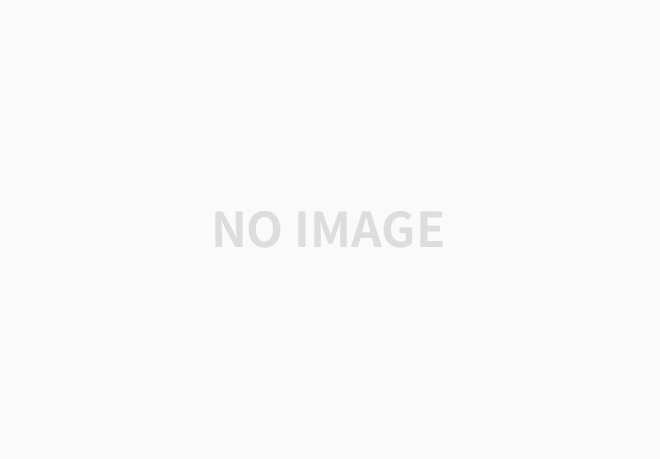
- 스프링 시큐리티의 경우 필터 체인은 다중으로 설정할 수 있음
- URL 패턴별로 원하는 대로 권한을 체크하는 등 별도로 구성이 가능함
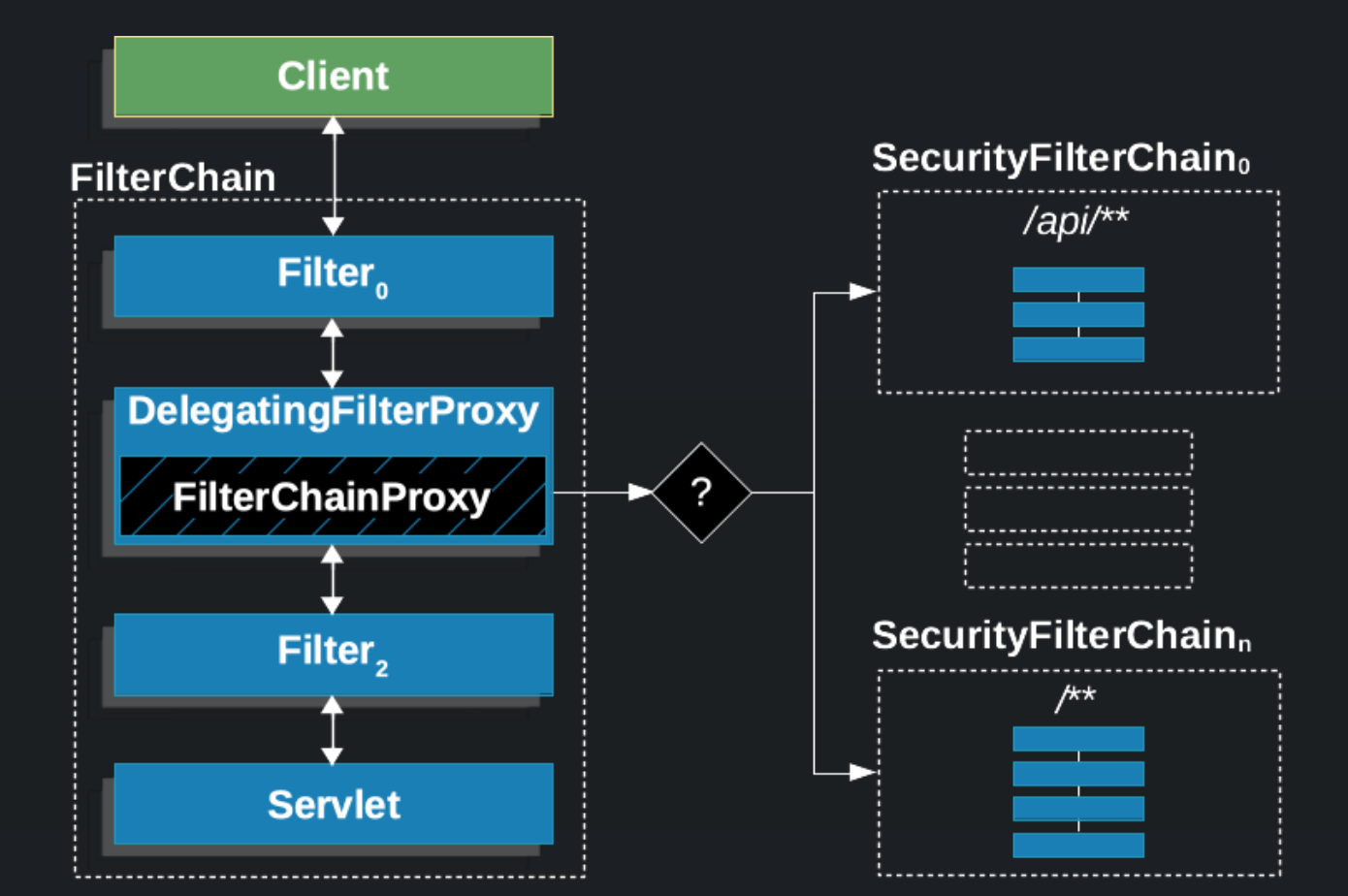
예시) 사용자 필터 추가한 SecurityCofing
package com.won.jwt.config;
import com.won.jwt.config.jwt.JwtAuthenticationFilter;
import lombok.RequiredArgsConstructor;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.config.annotation.authentication.configuration.AuthenticationConfiguration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.http.SessionCreationPolicy;
import org.springframework.security.web.SecurityFilterChain;
import org.springframework.security.web.authentication.www.BasicAuthenticationFilter;
import org.springframework.web.filter.CorsFilter;
import static com.won.jwt.code.AuthRole.*;
@Configuration
@EnableWebSecurity(debug = true)
@RequiredArgsConstructor
public class SecurityConfig {
private final CorsFilter corsFilter;
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http.csrf().disable();
http.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.addFilter(corsFilter)
.formLogin().disable()
.httpBasic().disable()
.addFilter(new JwtAuthenticationFilter())
.authorizeHttpRequests()
.antMatchers("/api/user/**").hasAnyRole(ADMIN.name(), MANAGER.name(), USER.name())
.antMatchers("/api/admin/**").hasRole(ADMIN.name())
.anyRequest().permitAll();
return http.build();
}
@Bean
public AuthenticationManager authenticationManager(AuthenticationConfiguration authenticationConfiguration) throws Exception {
return authenticationConfiguration.getAuthenticationManager();
}
}
위와 같이 시큐리티에 사용자 정의 필터를 추가해서 사용할 수도 있다. 참고할 수 있도록 JWT 설정을 위해 필터를 추가했던 config 코드는 위와 같다. config 파일 내에서 필요한 부분에 대한 세팅을 하면 사용할 수 있다.
필요한 경우 아래와 같이 위 코드에 추가해서 필터의 순서를 지정 할 수도 있다.
addFilterBefore 메서드의 경우 특정 필터 전에 전달 한 필터를 추가해 달라는 것이다.
아래와 같이 추가를 하게 되면 스프링 시큐리티 로그에서 아래와 같이 원하는대로 순서가 조절된 것을 볼 수 있다.
...
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http.addFilterBefore(new CustomFilter(), SecurityContextPersistenceFilter.class);
http.csrf().disable();
....
위와 같이 추가하는 경우 스프링 시큐리티 필터 체인은 아래의 순서로 세팅 된다.
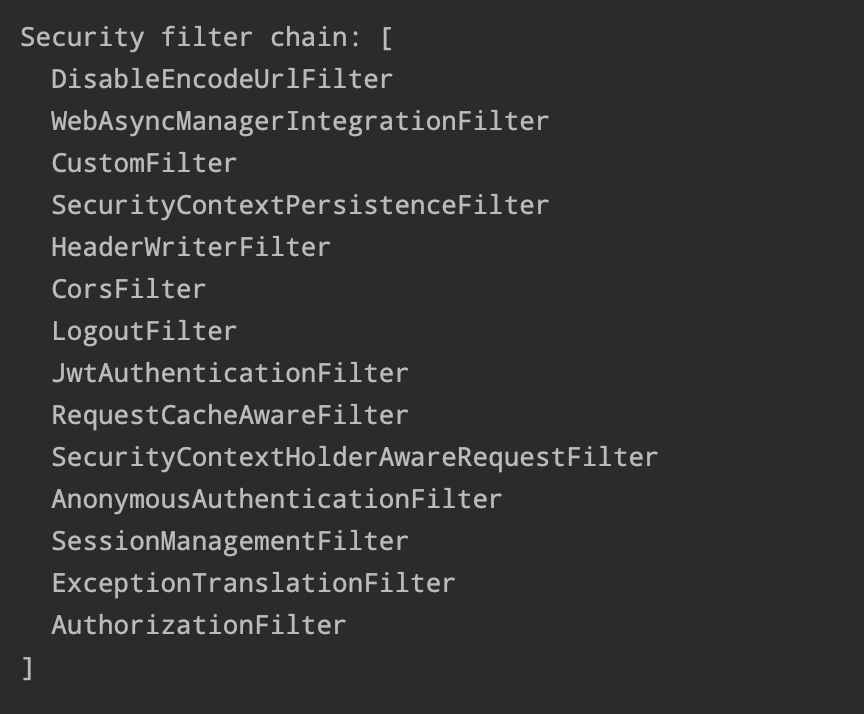
스프링 시큐리티 관련 로그를 보고 싶은 경우 SecurityConfig 클래스 파일에 (debug = true) 설정을 추가해 주면 된다.
@Configuration
@EnableWebSecurity(debug = true)
public class SecurityConfig {
...
}
Spring Security filter 종류
기본 스프링 시큐리티 필터 체인에 등록된 필터들은 아래와 같다
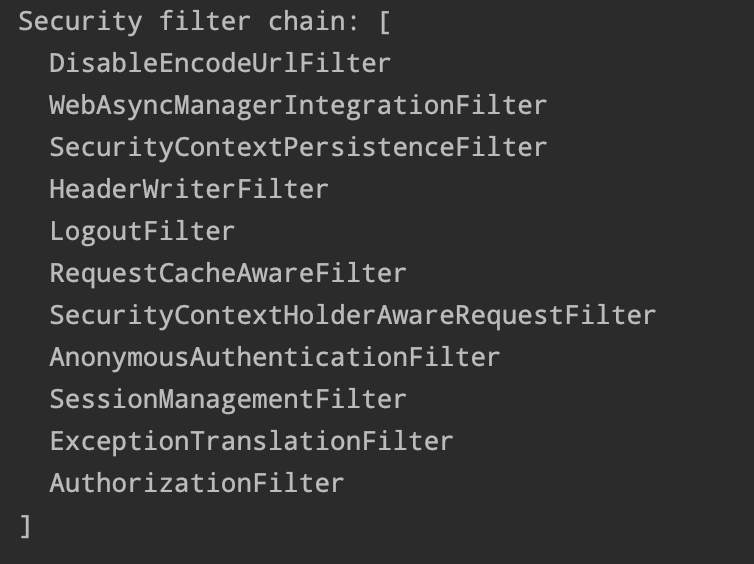
- DisableEncodeUrlFilter
- 세션 ID가 유출될 수 있으므로 URL에 포함되는 것을 막기 위해 HttpServeletResponse를 사용해 URL 인코딩이 되는 것을 막는 필터
- WebAsyncManagerIntegrationFilter
- 비동기 처리 시에도 SecurityContext 접근할 수 있도록 해주는 필터
- SecurityContext 통합관리
- SecurityContextPersistenceFilter
- 사용자 인증에 대한 정보를 담고 있는 SecurityContext 를 가져오거나 저장하는 역할의 필터
- HeaderWriterFilter
- 응답 Header에 spring security 관련 Header를 추가해 주는 필터
- LogoutFilter
- 설정된 로그아웃 URL로 오는 요청을 확인해 로그아웃 처리하는 필터
- RequestCacheAwareFilter
- 로그인 성공 후, 관련 캐시 요청을 확인하고 캐시 요청을 처리하는 필터
- 예) 로그인 하지 않고 방문했던 페이지 기억 후 로그인 후 해당 페이지로 이동 처리
- SecurityContextHolderAwareRequestFilter
- HttpServletRequest 정보를 감싸서 필터 체인 상의 다음 필터들에게 부가 정보 제공하기 위해 사용하는 필터
- AnonymousAuthenticationFilter
- 필터 호출 시점까지 인증되지 않는 경우 익명 사용자 전용 객체(AnonymousAuthentication) 만들어서 SecurityContext에 넣어주는 필터
- SessionManagementFilter
- 인증된 사용자와 관련된 세션 작업을 진행
- 유효하지 않은 세션에 대한 처리, 변조 방지 전략 등을 설정하는 작업을 처리하는 필터
- ExceptionTranslationFilter
- 요청을 처리하는 중에 발생할 수 있는 예외를 위임 또는 전달하는 필터
- AuthorizationFilter
- AuthorizationManager를 사용하여 URL을 통해 사용자의 리소스 접근에 대한 액세스를 제한하는 인증 필터
- 권한 부여 필터
반응형
'Java | spring > Spring Security' 카테고리의 다른 글
Spring Security 기본 개념 및 세팅 (+ please sing in 로그인 방법) (0) | 2023.10.01 |
---|
댓글